의료분야에서 데이터 학습시키기 파이썬 예제
2023. 12. 30. 06:53ㆍ카테고리 없음
의료 이미지 분석을 위한 딥 러닝 모델을 학습하는 과정에서는 데이터 준비, 모델 구성, 학습 및 평가 단계가 필요합니다. 아래에서는 예제로 사용할 간단한 데이터셋을 생성하고, CNN을 이용하여 학습하며, 학습된 모델을 H5 파일로 저장하는 예제를 제시하겠습니다.
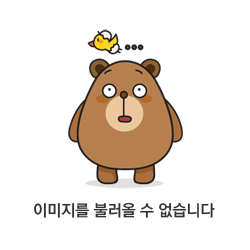
1. 데이터 준비
간단한 이미지 데이터셋을 생성합니다. 여기서는 두 개의 클래스(양성과 음성)를 가진 이진 분류 문제를 가정합니다.
import os
import cv2
import numpy as np
from sklearn.model_selection import train_test_split
from tensorflow.keras.utils import to_categorical
# 데이터 생성 및 저장 디렉토리
data_dir = 'path/to/your/dataset/'
os.makedirs(data_dir, exist_ok=True)
# 데이터셋 생성 및 저장
num_samples_per_class = 100
for i in range(2):
class_dir = os.path.join(data_dir, str(i))
os.makedirs(class_dir, exist_ok=True)
for j in range(num_samples_per_class):
img = np.random.rand(64, 64, 3) * 255 # 임의의 이미지 생성
img_path = os.path.join(class_dir, f'{i}_{j}.jpg')
cv2.imwrite(img_path, img)
# 이미지 데이터와 레이블 로드
X, y = [], []
for i, class_name in enumerate(os.listdir(data_dir)):
class_dir = os.path.join(data_dir, class_name)
for img_name in os.listdir(class_dir):
img_path = os.path.join(class_dir, img_name)
img = cv2.imread(img_path)
X.append(img)
y.append(i)
X = np.array(X)
y = to_categorical(y, num_classes=2)
# 데이터를 학습용과 테스트용으로 분할
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
2. 모델구성
간단한 CNN 모델을 구성합니다.
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Conv2D, MaxPooling2D, Flatten, Dense
# 모델 구성
model = Sequential()
model.add(Conv2D(32, (3, 3), activation='relu', input_shape=(64, 64, 3)))
model.add(MaxPooling2D((2, 2)))
model.add(Conv2D(64, (3, 3), activation='relu'))
model.add(MaxPooling2D((2, 2)))
model.add(Conv2D(64, (3, 3), activation='relu'))
model.add(Flatten())
model.add(Dense(64, activation='relu'))
model.add(Dense(2, activation='softmax')) # 출력 레이어는 클래스 수에 따라 조정
3. 모델 컴파일 및 학습
# 모델 컴파일
model.compile(optimizer='adam', loss='categorical_crossentropy', metrics=['accuracy'])
# 모델 학습
model.fit(X_train, y_train, epochs=10, batch_size=32, validation_data=(X_test, y_test))
4. 모델평가
# 모델 평가
loss, accuracy = model.evaluate(X_test, y_test)
print(f'Test Loss: {loss}, Test Accuracy: {accuracy}')
# 학습된 모델을 H5 파일로 저장
model.save('path/to/your/model.h5')
이 예제는 간단한 데이터셋과 모델을 사용하여 학습하고, 학습된 모델을 H5 파일로 저장하는 과정을 보여줍니다. 실제 의료 데이터셋과 복잡한 모델을 사용해야 하며, 데이터 전처리와 모델 구성 등을 더욱 정교하게 수행해야 합니다.